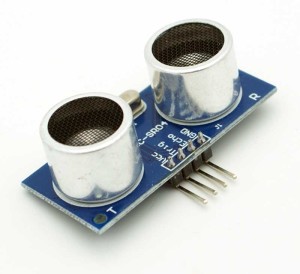
Arduino Ultrasonic Range Finder – HC-SR04
You can download our e-book ‘Learn Arduino’ from this link
In this tutorial we present a method of measuring the distance between an Arduino and nearby objects. It is of particular use for automated robots for giving them ‘eyes’ to look out for nearby objects, measure the distances between them, and acting upon that distance. We present the HC-SR04 range finder sensor
The cheap sensors normally have 2 cylindrical objects resembling a speaker with pins in the middle. Well, one of them is a speaker, the other is a microphone.
The sensor has 4 pins +5v, GND, Trig and Echo. Ignoring the obvious (+5v and the GND), we have the remaining 2 which are used to calculate the distance. The microcontroller pulls the Trig pin to High for 10 microseconds, then pulled down again. At this point the sensor sends an ultrasonic signal (around 40kHz I believe) from the speaker and waits for an echo. If an echo is picked by the sensor, the Echo pin is pulled down (being previously pulled up by the microcontroller). The time it takes from triggering the signal to the echo is the time taken for sound to travel from the sensor to the object and back.
Knowing the speed of sound which is roughly 340.29m/s we can calculate the distance traveled by sound in that time, then dividing it by 2 to get the distance from the object.
Sounds difficult? No problem, a library saves the day and makes everything easy.

Connecting the ultra sonic sensor to Arduino is super easy.
The library is called NewPing and can be downloaded here. Install the library. If you need help read here.
Now first things first. Wire up you sensor to the Arduino as follows
Arduino => UltraSonic Sensor
+5V => +5V
GND => GND
Echo => D11 (or any other pin)
Trigger => D12 (or any other pin)
You can use any pins you want for Echo and Trigger. Now open the Arduino IDE and copy the following code. This code is an example found in the NewPing Library. I will explain bits and pieces below.
#include <NewPing.h> #define TRIGGER_PIN 12 // Arduino pin tied to trigger pin on the ultrasonic sensor. #define ECHO_PIN 11 // Arduino pin tied to echo pin on the ultrasonic sensor. #define MAX_DISTANCE 200 // Maximum distance we want to ping for (in centimeters). Maximum sensor distance is rated at 400-500cm. NewPing sonar(TRIGGER_PIN, ECHO_PIN, MAX_DISTANCE); // NewPing setup of pins and maximum distance. void setup() { Serial.begin(115200); // Open serial monitor at 115200 baud to see ping results. } void loop() { delay(50); // Wait 50ms between pings (about 20 pings/sec). 29ms should be the shortest delay between pings. Serial.print("Ping: "); Serial.print(sonar.ping_cm()); // Send ping, get distance in cm and print result (0 = outside set distance range) Serial.println("cm"); }
The library makes it very easy to use this sensor as you can see from the code above.
The line ‘#define MAX_DISTANCE 200’ defines the maximum length we can read, in cm. Normally these sensors are rated between 400cm and 500cm, but I personally recommend a maximum of 200 cm or maybe 250cm. In practice I got fairly good results below 250cm but the percentage error increased as it reached 400cm. The line below is initializing a NewPing object named sonar with the pin values and the maximum distance.
NewPing sonar(TRIGGER_PIN, ECHO_PIN, MAX_DISTANCE);
The most important line is the ‘Serial.print(sonar.ping_cm());’, particularly ‘sonar.ping_cm()’. This function will do all the initialization sequence and calculations necessary to get the distance, and returns the length in cm.
If the returned value is ‘0’ then the distance is out of range … in this example, above 200 cm.
Now you are ready to install this sensor on your robot 🙂 In the mean time, you might want to take a look at another cool albeit advanced functionality of the NewPing library, which is the multi sensor example.
You will find it under NewPing15Sensors, and it shows how the library can be used to monitor 15 ultrasonic range finders at the same time!! Now your robot might need a vision upgrade!!
Buy ultrasonic sensors on (ebay)
Happy Building 🙂
Very interesting and very well explained. Few articles that I read from start till end!
You have inspired me to start to build a blog myself. You can follow me on http://brainyprojects.com/ some time later this year!